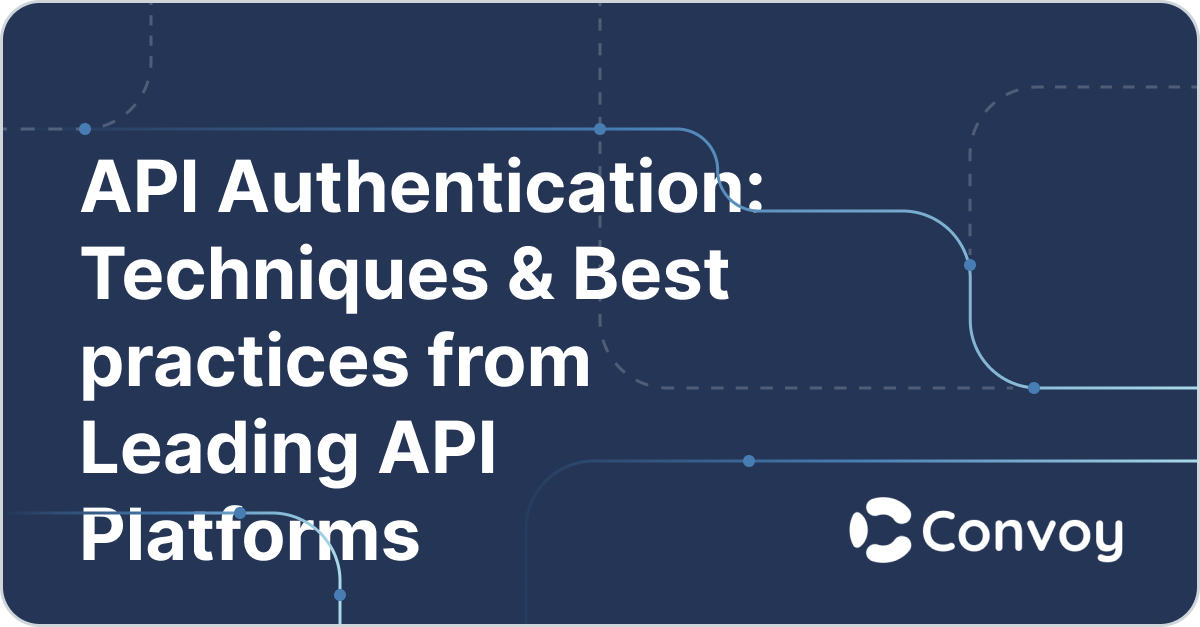
A large portion of the software market is API-driven, hence it makes sense that many startups and companies building new products want to implement APIs as a way for developers to interact or integrate with their services.
This is a series that will evaluate the mechanisms and best practices that leading API companies are using to build secure, scalable, and user-friendly APIs.
The first in the series will focus on API authentication.
There are multiple ways to implement API authentication. They are often one of these: Basic authentication, API key authentication, and token-based authentication. There are several forms of token-based authentication, the most common of which will be considered in this article.
In general, this article will explain what API authentication is, then expound on the mechanisms that existing successful API providers are using for their authentication, and what to consider when deciding the method of authentication to adopt based on your use case.
TL;DR
Most common Authentication methods and popular platforms using them.
API Keys: Google Maps API & Stripe, among others.
Personal Access Tokens: Github & Airtable among others.
OAuth: Spotify & X(twitter) among others.
Adoption of any of these techniques should depend on how your API will be used, and your specific security needs.
What is API Authentication
Simply put, API authentication refers to the act of restricting access to API resources to only trusted entities. To determine a trusted entity, an API server would expect an entity to meet certain criteria, such as possessing a valid credential. Authentication here is closely related to authorization, but their roles differ. API authorization would refer to a means of specifying what an entity or user can or cannot do with an API even after they have gained access to it.
The least common Auth Technique: Basic Authentication
HTTP Basic Authentication is the least secure among all the mechanisms for API authentication, hence it is the least adopted. This form of authentication involves collecting and storing the credentials, such as username and password of API consumers, and then requiring them to pass these credentials in the HTTP header for every API call made to the API server, these credentials are then checked for validity in order to authenticate the request.
This method of authentication although simple to implement, is insecure because the credentials are not encrypted and can be exposed during transmission especially if used without HTTPS.
Other forms of authentication such as API keys and Token-based authentication are more commonly adopted because of their improved security mechanisms in comparison to basic authentication, with token-based authentication being the most inherently secure.
API Keys
API Keys are yet another simple and straightforward method of authentication. They are unique alphanumeric strings generated by the API provider and given to the developers who register their applications to include in their requests. The API Keys then act as a passcode, allowing the API to identify and authenticate registered clients when they make a request.
Who is using it: Many API providers use API keys, including big players like Google Maps API and Stripe. What's common among successful API providers using API keys is that they implement other measures to help their consumers keep their API keys secure. For instance, the Google Maps API platform makes provision for consumers to restrict the usage of their API keys to only certain applications, or IP addresses. This way a stolen API key cannot be used unless the request is coming from within the bounds of the restriction.
Stripe equally implements a similar feature that allows one to restrict the IP addresses that can make API calls using an API key. In addition, they implement key Rolling. Rolling a key revokes it and generates a replacement key. Keys can be rolled manually or via a schedule that rolls the key after a certain time.
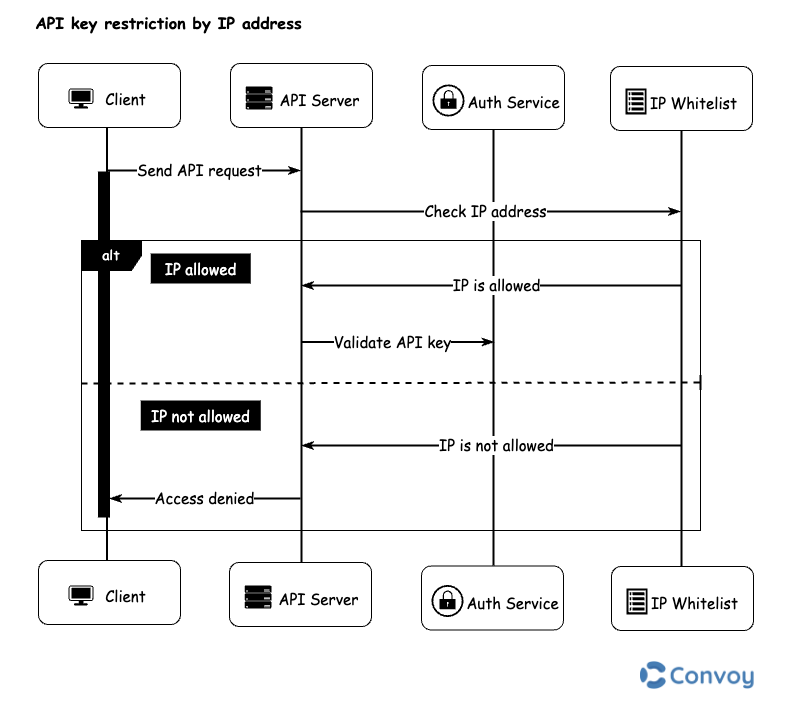
Should you use it: API keys are ideal for server-to-server communication but not for individual user authentication because they do not contain data for user-level identification, so consider if your use case fits this kind of communication model. Also, consider your security needs. API keys just like the credentials in HTTP basic authentication are not encrypted during transit hence you must always use them with HTTPS to enhance their security. With API keys though, it is also easier to build in other security measures such as the ability to roll the API keys and to restrict the keys to only certain projects, applications, or IP addresses.
Token-based authentication
Tokens are a proven means to securely authenticate and even authorize users and other principals, thus, they've become a popular choice for API authentication. The following are the most common forms of token-based authentication that work for APIs.
1. Personal Access Tokens
Personal Access Tokens (PATs) are randomly generated strings of characters used to securely authenticate API requests on behalf of a user. They are alternatives to passwords and are commonly used for accessing APIs and services that require user-level permissions, often in scenarios involving automation, scripts, or personal applications. Unlike API keys, personal access tokens are tied to individual user accounts and are delegated specific authorization levels over the API they're created for. They also come with expiry dates.
Who is using it: Companies such Github and Airtable among many others, use personal access tokens for API authentication. Some GitHub REST API endpoints do not require authentication, but to gain access to all the available endpoints and an increased rate limit, authentication via an access token is required. If access to the API is needed for personal use, (such as to grant access to a program) then the kind of token required is a personal access token.
In the same vein, Airtable uses personal access tokens to authenticate requests to the API when an integration is created for a user account. With the personal access token, a third-party program can act on behalf of the user account for which the token is created.
In general, personal access tokens inherit the permissions of the user account they're associated with. But what's common between Github and Airtable's PAT is that they do not simply inherit the permissions of the user they're tied to, instead, you can adjust the permission on the access token to include only what is necessary. GitHub currently supports both fine-grained personal access tokens and personal access tokens (classic).
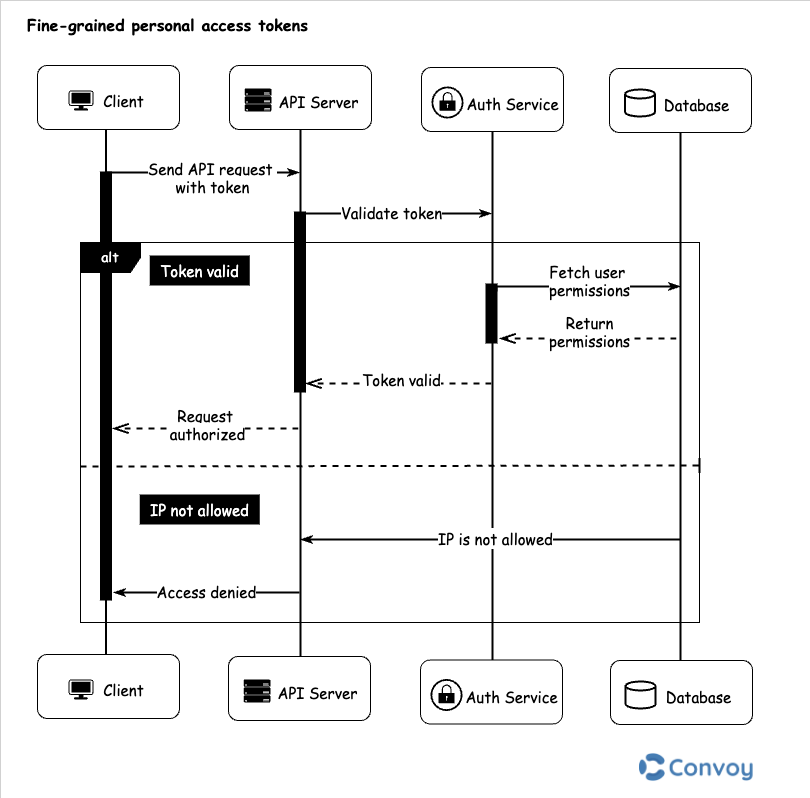
Should you use it: If your REST API serves individual user accounts that could have differing permission requirements, then personal access tokens might be ideal for your use case. Other things to keep in mind include that PATs are meant to be short-lived, hence you'll need to decide how long tokens can remain valid, whether they have a fixed lifespan or a user-defined lifespan, and a mechanism to regenerate, revoke, or delete access tokens.
consider also whether you may need to implement fine-grained personal access tokens, this feature is useful especially if your users sometimes need to grant third parties permission to call some of your API endpoints on their behalf.
2. OAuth
OAuth is an open standard for access delegation commonly used to grant websites or applications limited access to user information without exposing login details. OAuth supports several grant[https://oauth.net/2/grant-types/] types, which refer to the means or flows through which the apps or websites obtain an access token required to make calls to an API resource. Among them, the grant type "Authorization Code" grants permission to an app or website to act on behalf of a user, while the type "Client Credentials" is used by client apps to obtain an access token outside of the context of a user.
Who is using it: X and Spotify among many others use OAuth to authenticate their APIs. X uses the Authorization code grant type together with PKCE extension to authenticate Twitter developer apps, allowing them to make API requests on behalf of any Twitter account, as long as the user authenticates the app. PKCE is not a form of client authentication, rather it is a way for a client app to prove to the authorization server that the authorization code it's presenting is authentic, before exchanging it for an access token.
Spotify equally implements the Authorization code and Authorization code with PKCE extension grant types. The first is ideal for long-running applications like web and mobile apps where the user grants permission only once. For authorization in applications where the client secret cannot be securely stored, the authorization code type with PKCE is recommended.
Spotify also supports the Client Credentials flow for granting permission to endpoints that do not access user information.
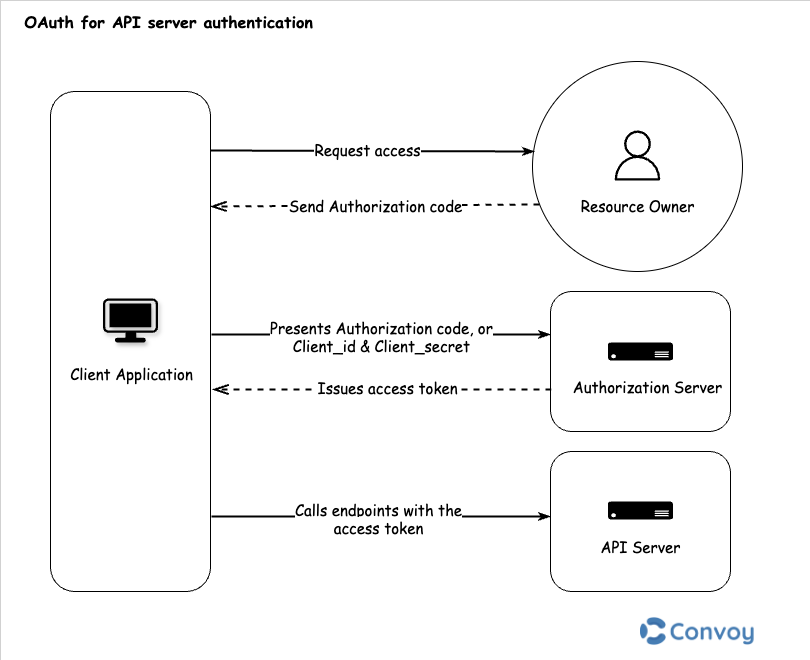
To remove the complexity around authenticating with the available authentication methods, twitter client libraries automatically handle the difficult part of API authentication for developers.
Should you use it: If your API requires fine-grained authorization access and will be consumed through a mobile app or a web app, then OAuth with the Authorization code grant type will be a great choice. Recall that making the PKCE extension flow available will be necessary if developers sometimes need to work with applications where the client secret cannot be securely stored.
If otherwise your API or some resources on your API do not require fine-grained authorization access, and a machine-to-machine communication model is fitting, then consider the Client Credentials Grant.
3. JWT
JWT-based access tokens are tokens formatted as JSON objects and contain all necessary information needed to authenticate and authorize a user or an app without the need for database lookups. A server issues this token after a user submits a means of identification (usually username and password) through a client, which the client includes in their requests when they try to access resources on the server.
Since JWTs are self-contained, they are especially suitable for REST APIs which are stateless in nature. However, they're not commonly used to authenticate public APIs, they're more suitable for internal APIs. This is because issuing a token via the JWT structure is not as straightforward as with obtaining a PAT, or with OAuth2 which mostly automates the process of obtaining an access token.
It's still possible to use JWTs even with public APIs by:
Creating an authentication page: with this, when a user signs in, a token is generated for them, which they could then download and use for their API calls.
Use OAuth2 together with JWT: You could have your OAuth2 authorization server issue JWTs as the access token, combining the strength of OAuth and the stateless nature of JWTs.authentication server.
What's next
In this first part of the series, you got to learn about how popular API platforms are implementing the most common API authentication techniques. Some of these techniques include API keys and token-based authentication methods such as personal access tokens and OAuth2. You saw some of the things to take into consideration when choosing an authentication type for your APIs.
No one authentication method is 100% secure, each method is simply another layer of security in addition to all other security best practices that you must adopt. It's not uncommon to implement more than one mechanism for authentication, choosing the best method for different parts of your API or for different entities or principals.
The next in the series will go in-depth on authorization. Hope to see you there!
Getting Started with Convoy
Already have an API, and want to send or receive webhooks from it? Get started in minutes at cloud.getconvoy.io/signup.